In the ever-expanding world of non-fungible tokens (NFTs), the ability to deploy your own NFT contract is a powerful tool. One such contract gaining popularity is the ERC721a contract, renowned for its efficiency and flexibility over its predecessor, the ERC721. If you’re a developer, creator, or tech-savvy enthusiast interested in creating NFTs, understanding how to deploy an ERC721a contract for NFT creation is invaluable. This guide will walk you through the process, highlighting important steps and best practices along the way.
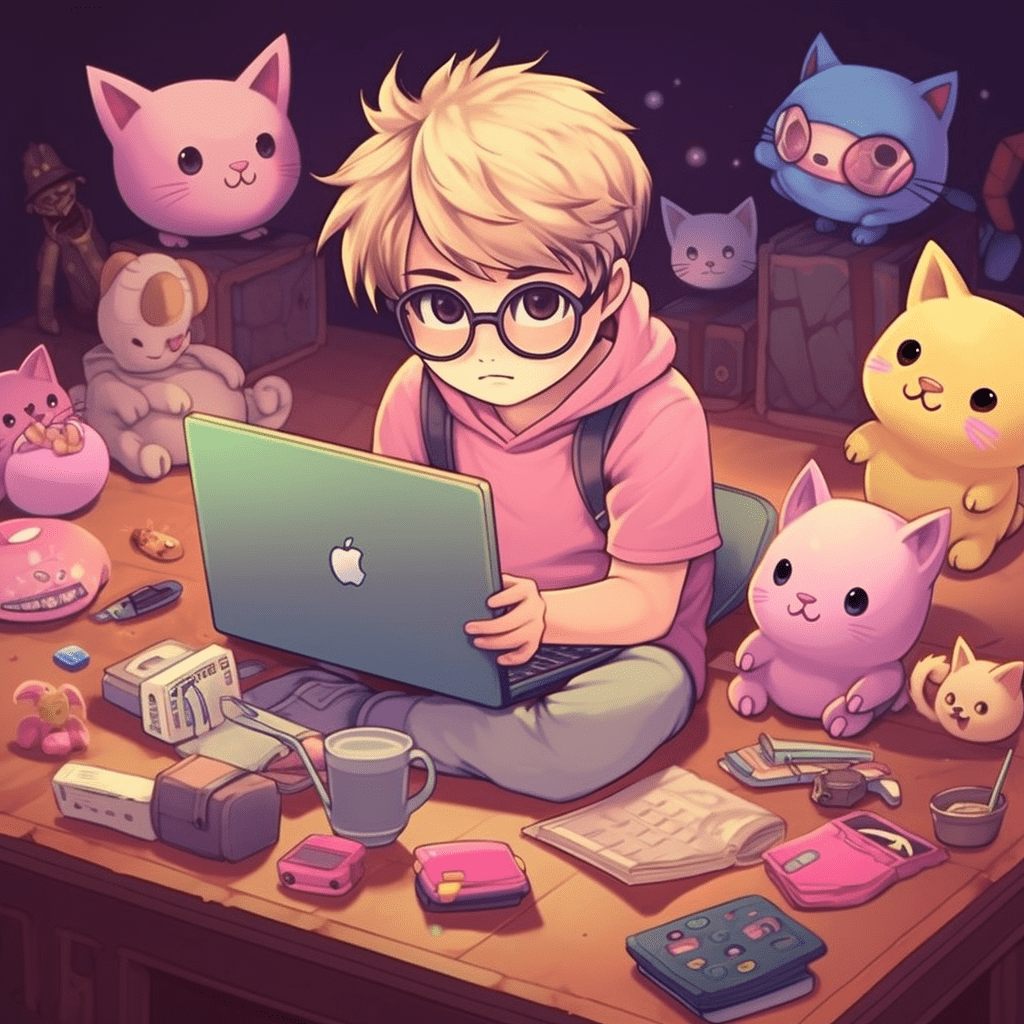
Understanding the Prerequisites
Before diving into the details of deploying an ERC721a contract, let’s look at the prerequisites you’ll need.
Familiarity with Solidity
Solidity is the programming language used for writing smart contracts on the Ethereum blockchain, including the ERC721a contract. A basic understanding of Solidity syntax and structures is essential for deploying an ERC721a contract.
Ethereum Development Tools
You’ll need access to Ethereum development tools such as Truffle, which is a popular development framework for Ethereum, and Ganache, a personal blockchain for Ethereum development. Another crucial tool is MetaMask, a browser extension that allows you to interact with the Ethereum blockchain.
An Ethereum Wallet
To deploy your contract on the Ethereum blockchain, you’ll need an Ethereum wallet with some Ether (ETH) in it to pay for the gas fees associated with contract deployment.
Deploying Your ERC721a Contract
With the prerequisites covered, let’s delve into the actual process of deploying an ERC721a contract for NFT creation.
Writing Your Contract
The first step in deploying an ERC721a contract is to write the contract itself. A basic ERC721a contract would include functions for minting tokens, transferring tokens, and managing token metadata.
Your contract should inherit from the ERC721a standard to ensure it adheres to the appropriate token standard and includes the necessary functions. Here is a basic example of what an ERC721a contract might look like:
pragma solidity ^0.5.0;
import "@openzeppelin/contracts/token/ERC721/ERC721Full.sol";
import "@openzeppelin/contracts/drafts/Counters.sol";
contract MyNFT is ERC721Full {
using Counters for Counters.Counter;
Counters.Counter private _tokenIds;
constructor() ERC721Full("MyNFT", "MNFT") public {
}
function mint(address recipient, string memory tokenURI) public returns (uint256) {
_tokenIds.increment();
uint256 newItemId = _tokenIds.current();
_mint(recipient, newItemId);
_setTokenURI(newItemId, tokenURI);
return newItemId;
}
In this code snippet, the mint
function allows for the creation of new tokens. The _mint
function, which comes from the ERC721 standard, is used to assign the newly minted token to an address, and _setTokenURI
is used to associate metadata with the token.
Testing Your Contract

Before deploying your contract to the Ethereum mainnet, it’s wise to test it first. This is where tools like Truffle and Ganache come in handy. You can write test cases for your contract in JavaScript using Truffle and run these tests on your local Ethereum blockchain provided by Ganache.
Deploying Your Contract
Once you’re satisfied with your contract and its tests, you’re ready to deploy it to the Ethereum mainnet. Deploying the contract involves sending a special transaction to the Ethereum network that includes the compiled bytecode of your contract and any constructor parameters.
In Truffle, this can be achievedby writing a migration script. Here’s a simplified example of how to do it:
const MyNFT = artifacts.require(“MyNFT”);
module.exports = function(deployer) {
deployer.deploy(MyNFT);
};
After running the migration script, your ERC721a contract will be live on the Ethereum network, ready for interaction.
Interacting with Your Contract
After deployment, the next step is to interact with your contract to mint your NFTs. You can write a script using the web3.js library, which provides a collection of libraries that allow you to interact with a local or remote Ethereum node using HTTP, IPC, or WebSocket.
Here’s an example of how you can use web3.js to interact with your deployed contract:
const Web3 = require(‘web3’);
const MyNFT = require(‘./build/contracts/MyNFT.json’);
const init = async () => {
const web3 = new Web3(‘https://mainnet.infura.io/v3/YOUR-INFURA-KEY’);
const id = ‘1’; // The id of your NFT
const metadata = ‘https://my-nft-api.com/token/’ + id; // The metadata of your NFT
const accounts = await web3.eth.getAccounts();
const myNFT = new web3.eth.Contract(
MyNFT.abi,
MyNFT.networks[MyNFT.network_id].address
);
await myNFT.methods.mint(accounts[0], metadata).send({ from: accounts[0] });
};
init();
by writing a migration script. Here’s a simplified example of how to do it:
javascriptCopy codeconst MyNFT = artifacts.require("MyNFT");
module.exports = function(deployer) {
deployer.deploy(MyNFT);
};
After running the migration script, your ERC721a contract will be live on the Ethereum network, ready for interaction.
Interacting with Your Contract

After deployment, the next step is to interact with your contract to mint your NFTs. You can write a script using the web3.js library, which provides a collection of libraries that allow you to interact with a local or remote Ethereum node using HTTP, IPC, or WebSocket.
Here’s an example of how you can use web3.js to interact with your deployed contract:
javascriptCopy codeconst Web3 = require('web3');
const MyNFT = require('./build/contracts/MyNFT.json');
const init = async () => {
const web3 = new Web3('https://mainnet.infura.io/v3/YOUR-INFURA-KEY');
const id = '1'; // The id of your NFT
const metadata = 'https://my-nft-api.com/token/' + id; // The metadata of your NFT
const accounts = await web3.eth.getAccounts();
const myNFT = new web3.eth.Contract(
MyNFT.abi,
MyNFT.networks[MyNFT.network_id].address
);
await myNFT.methods.mint(accounts[0], metadata).send({ from: accounts[0] });
};
init();
In this example, an instance of the contract is created using the ABI and the contract address. The mint
function is then called to create a new NFT with the specified metadata and owner.
Conclusion: Launching Into the NFT Space with ERC721a Contracts

Deploying an ERC721a contract for NFT creation may seem complex, but with the right tools and guidance, it can be an exciting journey into the heart of the Ethereum ecosystem. By mastering this process, you open the door to a realm of possibilities in the dynamic world of NFTs.