
Creating non-fungible tokens (NFTs) has never been easier, thanks to the emergence of new token standards like ERC721a. As a successor to the ERC721 standard, ERC721a offers several improvements, such as reduced gas costs, batch operations, and dynamic metadata updates. In this article, we’ll guide you through the process of creating an ERC721a contract for your NFT project. This tutorial assumes you have a basic understanding of Ethereum, smart contracts, and the Solidity programming language.
Set Up Your Development Environment
Before you start coding your ERC721a contract, you’ll need to set up your development environment. Here are the essential tools you’ll need:
- Node.js and npm: Install the latest versions of Node.js and npm (the Node.js package manager) on your computer.
- Truffle: Truffle is a popular development framework for Ethereum. Install it globally using npm by running
npm install -g truffle
. - Ganache: Ganache is a personal blockchain for Ethereum development. Download and install the Ganache GUI or the Ganache CLI, depending on your preference.
- Metamask: Metamask is an Ethereum wallet and browser extension that allows you to interact with decentralized applications (dApps). Install the Metamask browser extension and set up an account.
Initialize Your Truffle Project
Once you have your development environment set up, it’s time to create a new Truffle project. Open your terminal and follow these steps:
- Create a new directory for your project and navigate to it:
mkdir MyNFTProject && cd MyNFTProject
- Initialize a new Truffle project:
truffle init
- Open your project folder in your favorite code editor.
Install the ERC721a Dependencies
To create an ERC721a contract, you’ll need to use the OpenZeppelin Contracts library, which provides a collection of reusable Solidity contracts, including the ERC721a implementation. Install the library using npm:
- Initialize a new npm project:
npm init -y
- Install the OpenZeppelin Contracts library:
npm install @openzeppelin/contracts
Create Your ERC721a Contract
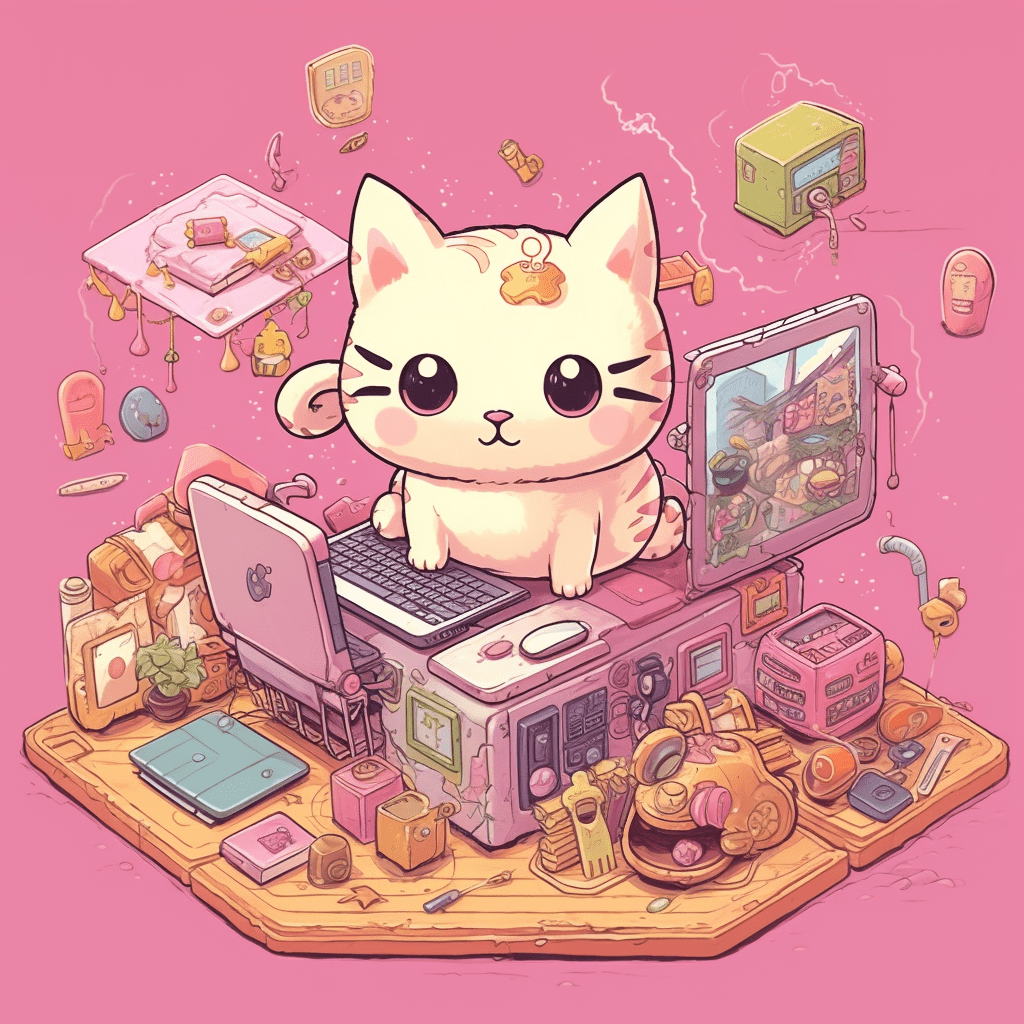
Now you’re ready to create your ERC721a contract. Follow these steps:
- Create a new Solidity file in the
contracts
folder of your Truffle project. You can name itMyNFT.sol
. - Import the ERC721a contract from the OpenZeppelin library and create a new contract that inherits from it. Your code should look like this:
pragma solidity ^0.8.0;
import “@openzeppelin/contracts/token/ERC721/extensions/ERC721a.sol”;
contract MyNFT is ERC721a {
constructor() ERC721a(“MyNFT”, “MNFT”) {
}
}
This code defines a simple ERC721a contract with a name (MyNFT
) and a symbol (MNFT
).
- If you want to add any custom functionality to your contract, you can do so by extending the
MyNFT
contract. For example, you could add a function to mint new tokens:
function mint(address to, uint256 tokenId) public {
_mint(to, tokenId);
}
Deploy Your Contract to a Local Blockchain
Before deploying your contract to the Ethereum mainnet, it’s a good idea to test it on a local blockchain. Start Ganache, and make sure it’s running on the same network as your Truffle project (by default, both use the same network configuration). Then, add a migration file for your contract in the migrations
folder. Name it 2_deploy_myNFT.js
and add the following code:
const MyNFT = artifacts.require(“MyNFT”);
module.exports = function (deployer) {
deployer.deploy(MyNFT);
};
Next, update your truffle-config.js
file to use the correct network and compile settings. Then, deploy your contract to the local blockchain by running truffle migrate
.
Test Your Contract
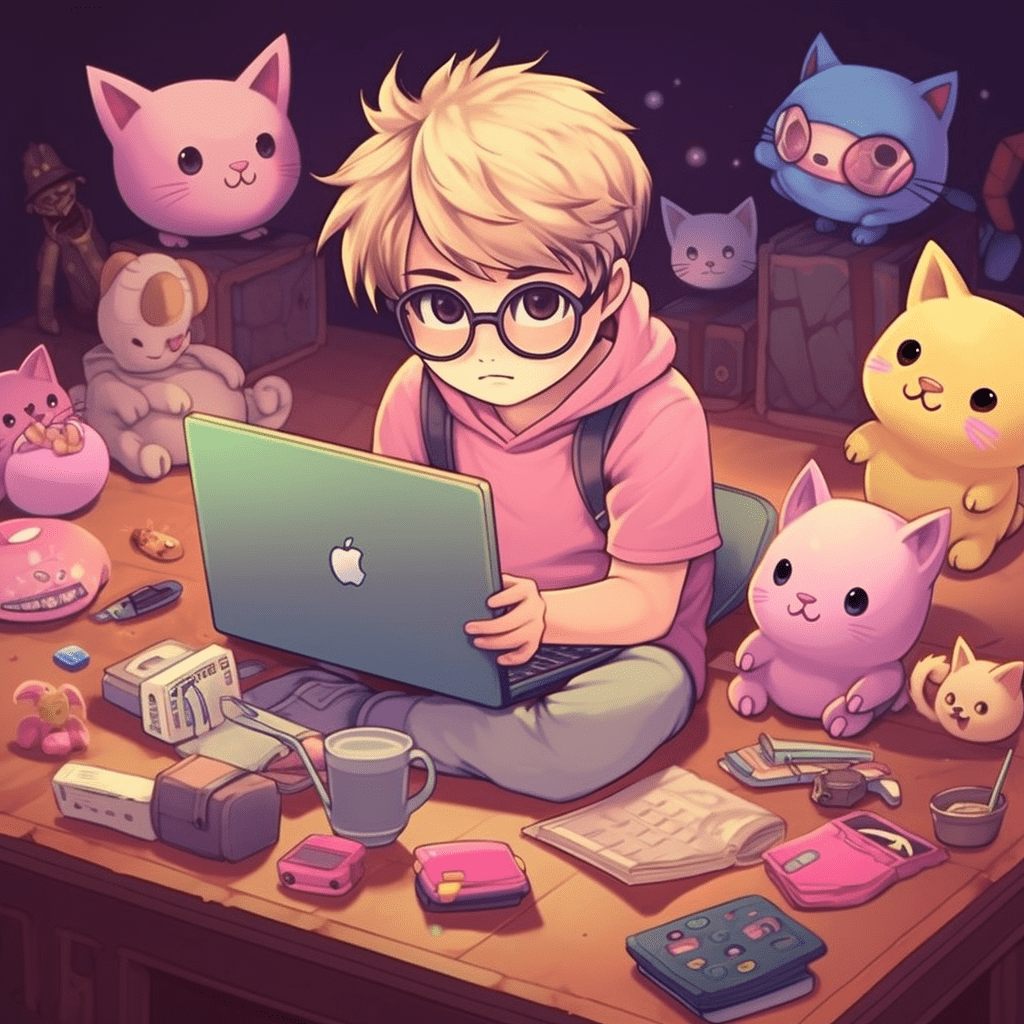
Testing your contract is an essential step to ensure it works as expected. Create a new file in the test
folder named myNFT.test.js
. Use JavaScript and the Truffle testing framework to write tests for your contract’s functions. Here’s an example test for the mint
function:
const MyNFT = artifacts.require(“MyNFT”);
contract(“MyNFT”, (accounts) => {
let myNFT;
beforeEach(async () => {
myNFT = await MyNFT.new();
});
it(“should mint a new token”, async () => {
const tokenId = 1;
const to = accounts[1];
await myNFT.mint(to, tokenId);
const owner = await myNFT.ownerOf(tokenId);
assert.equal(owner, to, "Token was not minted correctly");
});
});
Run your tests using the truffle test
command.
Deploy Your Contract to the Ethereum Mainnet or Testnets
Once you’re satisfied with your contract’s functionality and have thoroughly tested it, you can deploy it to the Ethereum mainnet or a testnet. Update your truffle-config.js
file with the appropriate network settings and deploy your contract using the truffle migrate --network <network_name>
command.
Interact with Your Contract
After deploying your contract, you can interact with it using various tools, such as the Truffle console, web3.js, or a frontend application.
Final Thoughts

Creating an ERC721a contract for your NFT project can be a rewarding experience. By following this guide, you should now have a better understanding of how to set up your development environment, create and test your contract, and deploy it to the Ethereum network. Keep in mind that this tutorial serves as a starting point, and you can always add more advanced functionality to your contract as needed.